29 Nov 2017
If I'm starting a new iOS project, which language should I choose?
I’ve been crazy busy over the last few months - a good problem to have, but it means I’ve had no time to blog on here 🙁
I’ve been switching between loads of different technologies to build mobile apps during that time. I thought it might be interesting to share my experiences switching between Objective-C and Swift, and the pros and cons of each.
Objective-C
It’d been a while since I’d worked in Objective-C, but I took on a project for one of my clients to enhance and modernise a couple of their apps which hadn’t been touched in a while.
To be honest the original code was pretty awful - they’d been let down by some contractors in the past and it took me a while to get my head around how the app worked.
Objective-C is notorious ugly to look at, with all those square brackets in the method calling, but once you get used to it I find it pretty productive to use.
I think knowledge of iOS APIs is by far the most important thing when developing on iOS, not the particulars how to type the code to call the API methods required.
The biggest plus point for Objective-C is the speed and stability of Xcode and the compiler. After mostly doing Swift development in the last year or so, it was really nice to get fewer mysterious compiler problems.
I strongly suspect Apple still do most of their internal development using Objective-C, and until they move over wholesale to Swift there will be less internal pressure to improve Xcode to handle Swift more reliably.
The main downside was that more and more of the code examples out there on the web - especially for the more modern APIs - are now in Swift. Less opportunities for “Stack Overflow copy-and-paste” development 😊
Swift
Most of my own apps are written in Swift now, and I’ve been doing the usual summer updates to keep them up to date for iOS 11 - and this year also for the challenge of iPhone X and the notch.
I must admit I do like Swift, and in particular the way it forces you to think hard about nullability of objects. This enforced type safety can be a bit painful at times (all the “!” and “?”s) but I trulythink you end up with more reliable code in the end.
However, as I mentioned above, Xcode and the compiler are MUCH less reliable when using Swift. Quite often I’ve seen it do a poor job of recompiling changes, so you’re never 100% clear if you are seeing the latest code in the emulator. A restart of Xcode usually fixes it, but that’s a real productivity hit.
The Swift compiler is also feels noticeably slower than the Objective-C compiler. All those small delays soon add up.
Also Swift is still a bit of a moving target, and once again we have Swift 4 and changes to the language. These seem to be getting fewer over releases, but I really hope the language gets to some sort of stability soon.
So which language will I choose for new projects?
I think I’ll still choose Swift for any new projects when I have a choice. Apple have strongly signalled this is their preferred language going forward, and as a language I do prefer it to Objective-C.
However after a few months back using Objective-C, I’ve really enjoyed the stability and speed of the tools. Let’s hope the Swift tools can improve to a similar state soon.
26 Nov 2017
Daily Optimiser is the first iOS app I ever wrote, and it’s been looking a little tired and out of date recently.
So last week I spent a few hours improving and modernising the UI, and I’m quite pleased with the results (there are some screenshots are at the end of the article).
I’ve tried to be more consistent and subtle with the use of font size and color to give the information a clear hierarchy.
What was more interesting was how much I’ve learnt in the last few years of doing iOS development. Looking back at the original code, it’s clear I was I had no clear idea how best to structure the code. I was also very inexperienced in autolayout, and used tables EVERYWHERE to build screens!
I’ve also made the app iOS11 only to take advantage of large titles in the UI, but from my analytics I can see hardly anyone is using the app any more, so it’s not going to aggravate a non-existent user base.
I must admit I don’t actually use Daily Optimiser, as I prefer Todoist over Reminders as my task organiser. However if you are looking for a simple app based around Calendars and Reminders to help you organise your day, why not give it a go?
Screenshots of Daily Optimiser 4.0
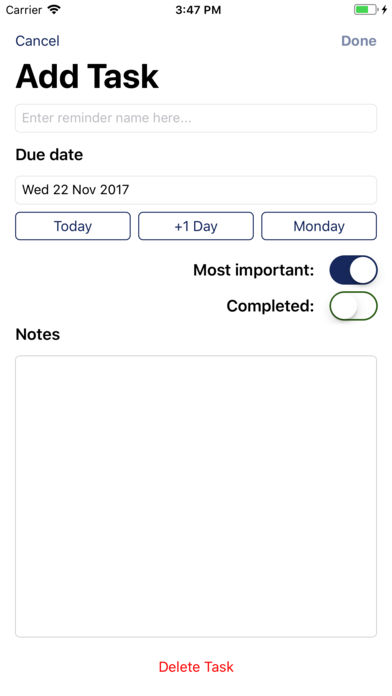
12 Feb 2017
Where I try to justify - mainly to myself - why it's OK to put ads in 'Count The Days Left'
I recently released a version of my Count the Days Left app which included ads for the first time.
I thought long and hard before doing this, so I thought it might be interesting to document how I worked through this decision, as it’s pretty typical
of the trade-offs independent software developers have to make.
How I make money
I’ve been a commercial software developer for nearly 30 years now (wow!), including over 10 years working at Microsoft (MSN/Bing/Skype).
Right now, I split my time between:
- Contracting out my skills to businesses for fixed-term projects
- Building mobile apps and websites for businesses
- Developing my own iOS and Android apps for both pleasure and profit
In 2017 I’m trying to diversify my business more, so the 3 areas above contribute more equally to the bottom line.
Currently, by far the biggest part of my income is working on contracts for other people,
and I’m trying to generate more independent streams of income so I can be more in control of both my time and where I work.
Why I built Count The Days Left
I built the app for a few reasons, but mainly:
- I wanted to showcase my skills to generate potential business
- I wanted this app for myself, and most of the alternatives in the App Store were either ugly or not quite what I wanted
For the first point, I’ve blogged extensively about how I’ve built the app,
as well as open sourcing the code on GitHub so potential customers can see my work.
The app has been pretty successful in meeting these aims, and has definitely helped in generating business (although it’s hard to measure the actual monetary value of this).
As you’d expect from a simple and niche app, it doesn’t have masses of users - daily users are definitely in the hundreds rather than the thousands.
In fact, some of the features (showing the days left as an icon badge, the Today widget, plus the Watch complication and app) are designed so the users don’t actually need to open the app every day, but I’m pretty proud of how it’s turned out.
Monitising the app
For any iOS app, there are three main ways of making money from an app:
- Charging to buy the app
- Adding an in-app purchase or subscription, probably to unlock additional functionality in an otherwise free app
- Adding ads
Now, unfortunately I suspect there is very little chance I’d sell many copies if I decided to charge for the app.
There are so many free competitors to this particular app, and even if my app is better than them - and obvioiusly I think it is :) - it’s very hard to
see how many customers would agree.
People are now so used to getting high-quality free software, generally from venture capital backed companies who are happy to lose lots of money
to capture large audiences in the hope of monitising that audience later. That makes it a very hard sell to convince people even to spend $0.99 on an app that
might provide them with lots of value.
Personally I’m happy to pay for good software, especially from independent software developers who are doing great work.
However I completely understand why most people
don’t think in that way, and as I can’t see the situation ever changing, for most consumer apps I can’t see there’s much point in even charging a very small amount.
Now this particular app only really does one thing (although hopefully it does it well!), so I can’t see any sensible way of adding “pro features”
to be unlocked by an in-app purchase.
I guess I could try a patronage/shareware model, and ask for money to support the app?
I’m not sure that would actually generate any money without some sort of persistent nagging which I don’t think I’d be happy with.
This probably gets to the nub of my problem with all of this discussion. I’m not sure if it’s a British thing, but the conflict between needing to get paid and
somehow seeming to be only doing it work the money is complicated.
I do want people appreciate and enjoy the app, and somehow this should be separate from the dirty business of getting paid.
So this just leaves adding ads.
Tasteful Ads?
I didn’t want the app’s main screen to show ads at any time. I think its’ main selling point is it’s a tasteful and good-looking app
(especially compared to some of my competitors), and slapping an ad there would completely negate that.
Therefore only other place left to put the ads is on the settings page, where you change the title, start and end dates of what you’re counting down to.
I’m reasonably happy with this compromise, although from a pure money-making point of view this page is not viewed very often, as the users only go thereevery time they start a new countdown.
I’m using Google to provide the ads via AdMob, and have customised them to be text-only and in a color that matches the rest of the app. At least in that way they are not too
jarring, and fit in as well as I can make them.
As you can see from this example, the way the ad looks is OK (even though the content is frankly a bit shit)
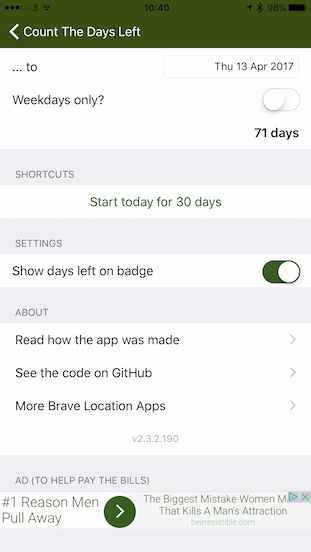
Is it worth it?
In the first week, I’ve made a few pennies - slight more than I expected, which was very little be honest!
However, I’m generally happy I’ve done this. I think it’s important all software developers get paid fairly for their work,
so turning the tide against the idea all software should be free - even the the smallest way - is OK.
Probably.
Obviously giving Google even more data is not “free” for my customers, as they are now paying for the app with their information.
I’m not 100% happy doing that, but as I’ve hopefully explained here, it’s a complicated trade-off.
Feel free to contact me via Mastodon at @johnp@bravelocation.com if you have any thoughts on any of this.
27 Jan 2017
Version 2.3 of Count The Days Left has now hit the App Store for your viewing pleasure.
I finally got around to upgrading the code to Swift 3. The Xcode migration code did a reasonable job with the syntax changes, but missed a few things around interface changes - especially in the WatchOS code - that
I had to fix manually.
I also fixed a very minor logic error in the date calculation in the settings page. Quite amazing it had been sitting there in plain sight for a long time without me noticing it.
Not much more to do in the app right now, but maybe WWDC in the summer will bring some exciting new areas in which to play in?
01 Jan 2017
All about how I'm blogging efficiently from my iPad Pro
Why do this?
Like most people who are trying the ‘iPad Work Lifestyle’, half the fun of trying it is the challenge to doing work a in different way. At times, working on the iPad certainly makes you think about how to solve problems to do the ‘simplest things’. Tasks that are natural on PCs may involve lateral thinking to complete on iOS.
It’s not all bad though. Single focus apps (or at most 2 in split screen view) do make it easier to concentrate on the task in hand. Also many apps are exceedingly well designed to do a few things very well rather than more general purpose PC apps.
Most of all, it somehow feels much more fun to write this on my iPad that it ever would on either my Mac or my Windows PC. I can’t really define why (novelty perhaps?), but it just does.
Right now, for me it’s all an experiment for 2017, so we’ll see how I feel later in the year if I keep this up.
Hardware
My iPad is a 2016 9.7 inch Pro model, and I love it. The display is truly outstanding, and it’s a really nice mix of relative power and portability.
I’m currently using an Amazon Basic Bluetooth Keyboard that I’ve had for a while (not sure it’s still available). It’s really light and easy to toss in my bag if I’m going out and about.
The keyboard does the job, but am seriously considering investing in either an Apple or Logitech Smart Keyboard Cover at some point, mainly for convenience. They’re not cheap though, so not sure right now if they’re worth it.
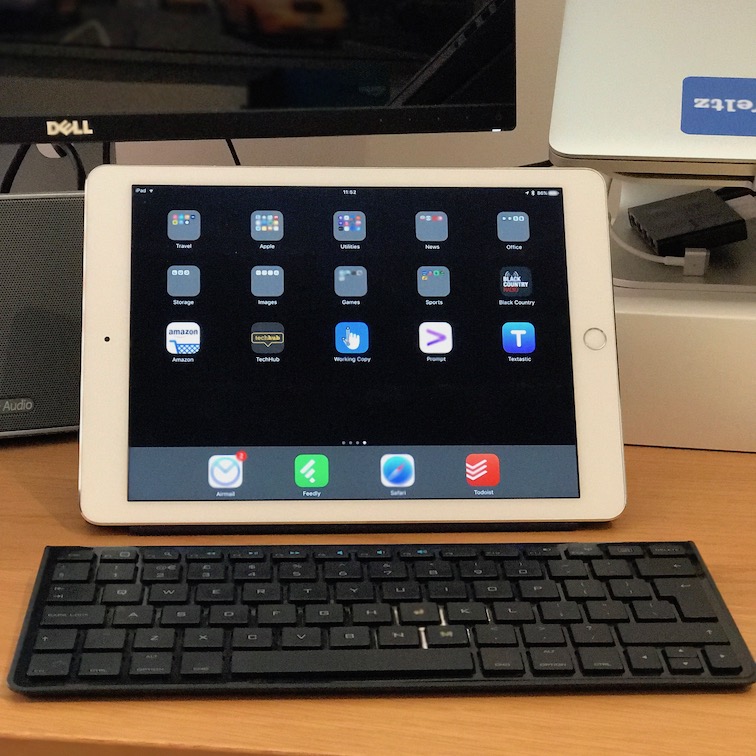
Hosting
Jekyll static site
I run quite a few of my sites on Jekyll, a static site generator popularised by GitHub. It’s pretty powerful (runs on Ruby), fully customisable, cross-platform, and is great for blogging in Markdown.
I like Jekyll anyway, but it makes it a good fit for this blog as it means I can write posts in Markdown and not have to worry too much about running some sort of web server on the iPad.
Digital Ocean
I’ve been using Digital Ocean for a year or so, and are really liking the simplicity of their setup and excellent documentation and support.
My virtual server is running Ubuntu 16.04, and I’m using NGINX as the web server pointing to the static site.
You need to be happy with the UNIX command line to setup the server, but I’m reasonably proficient and it’s much better (for me) to have full control over my server rather than being on a cheap shared host somewhere.
GitHub
The code for the site is all held in GitHub, and actually could be hosted from there as GitHub repositories can natively serve Jekyll sites.
This is really useful as I can test any changes after checking them in (via https://bravelocation.github.io/writingontablets.com/ if you are interested too!). This is really handy to check everything works before making the changes go live, as the preview of images etc. doesn’t work great in Textastic (see below)
Let’s Encrypt
I wanted to make this site HTTPS from the start - for both security and for Google ranking - and on my setup it’s easy to do this through Let’s Encrypt.
Let’s Encrypt offer free certificates for your web server, and can be kept up to date through automation. It’s a great service, and no-one has any excuse nowadays for not offering a secure site to thier customers.
Software
Working Copy (for Git)
As mentioned above, using GitHub is central to how my blogging setup works. Working Copy is a really nice (and highly recommended) Git client for iOS.
As well as the obvious Git functions, it also works as a document provider making it easy to open files in your repository in other programs.
Textastic (for text editing)
Textastic is a powerful multi-language code editor for iOS.
It has syntax highlighting for multiple languages (useful for me as I will not just be writing this blog on my iPad), and has a well thought out extended keyboard perfect for coding.
The built-in markdown preview is also pretty decent too, which helps a lot during the initial editing of posts.
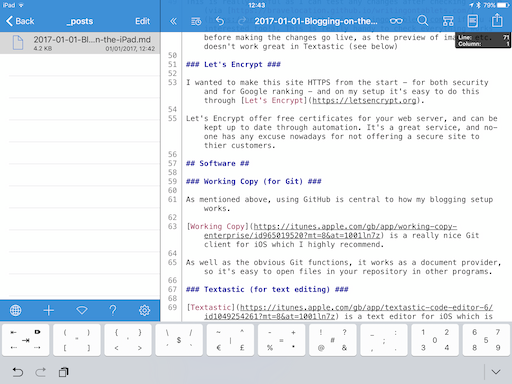
Image editing
I’m still working on the best solution for cropping and editing images ready for the site.
Right now I’m using screenshots direct from the iPad, or photos taken on my iPhone and cropping them using the built-in editing features in Photos.app, but that means the images are too large for the web site (even though the CSS handles the resizing well).
I suspect I’ll build some Workflows (see below) to handle the resizing part, but I haven’t done that yet.
Workflow
I think most people who use their iPad for more than just content consumption will know about Workflow,
the indepensible app for iOS automation.
I’m sure I’ll write more about how I’m using Workflow to simplify many tasks I need to do, but right now I have a script I use for releasing any changes to the site.
My process for publishing a new post is as follows:
- Write the new post in markdown
- Check the changes into Git, and push them up to GitHub
- Check the post looks OK on the GitHub-hosted site
- Log into the production server (via SSH), pull the latest changes from GitHub and then
jekyll build
the updated static site to generate the latest changes.
I have a nice Workflow that utilises the SSH action, which means I can automate the final point without having to actually use an SSH client
(I can use Prompt 2 if I actually need full SSH access).
The code in the ‘Run Script over SSH’ action loooks like this:
source .bash_profile
cd websites/[Directory]
git pull
/usr/local/rvm/gems/ruby-2.2.1/wrappers/bundle exec jekyll build
Note [Directory] is a variable I set earlier in the workflow from a multiple choice list, so I can reuse this script for my other sites hosted on my server.
I had to add in the source .bash_profile
(and the full path to the ruby bundle command) to get this to work reliably, but it’s a really quick way of pushing changes out with very little work.
Summary
So far, using the above process is working pretty well, and other than optimising the images I haven’t had to revert to using my Macbook Pro to do anything yet. Hopefully I’ll find an efficient way of handling images too soon.
It also means I can work on the site from anywhere, as it’s almost inconceivable I’ll be somewhere without either my iPad or my iPhone. That would be weird!
If you have any comments, improvements or suggestions, let me know on Mastodon at @johnp@bravelocation.com