Swift and Build Odds and Sods
22 Feb 2015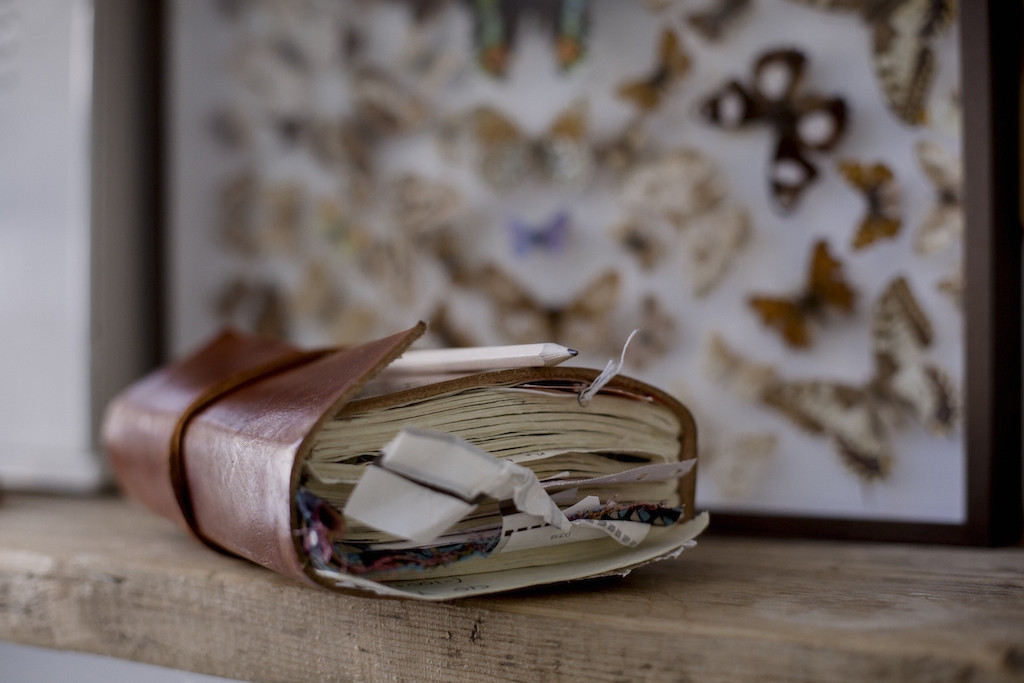
Lots more tweaks of the UI, in particular making a better Settings page.
It’s a pretty straightforward static table view, but there were a few things I learnt/remembered along the way that are worth sharing here.
Selectors in Swift
Installing the app on my iPad exposed a bug I’d introduced in a selector - just shows you can’t always believe everything you read on StackOverflow!
Setting up the handler for incoming notifications, you need to add a colon to the end of the method name in the selector e.g.
NSNotificationCenter.defaultCenter().addObserver(self, selector: "updateKVStoreItems:", name: NSUbiquitousKeyValueStoreDidChangeExternallyNotification, object: store)
Also on the function being selected, you need to annotate the function with @objc
so it can correctly interact with the Objective-C library code calling it
Custom data entry for text
I wanted a simple way of entering dates, as I stated in an earlier post simply adding a DatePicker to the page is pretty ugly and takes up a lot of screen.
The solution is to show the dates in a textbox, but then setting the inputView of the text box to be a DatePicker.
// Setup the date pickers as editors for text fields
self.textStart.inputView = self.startDatePicker
Obviously you have to take care in calling self.view.endEditing(true)
as appropriate when other elements are selected, but it’s a neat solution that works well.
Setting the build version automatically
I can’t remember where I found this originally, but I’ve used it in projects before. Basically I want to set the build number automatically based on the Github revision number, so I can call it in the code.
The trick is to add this script as part of the “Run Script” you can set in a target’s Build Phases
git=`sh /etc/profile; which git`
appBuild=`$git rev-list --all |wc -l`
/usr/libexec/PlistBuddy -c "Set :CFBundleVersion $appBuild" "${TARGET_BUILD_DIR}/${INFOPLIST_PATH}"
echo "Updated ${TARGET_BUILD_DIR}/${INFOPLIST_PATH}"
You can then use this to add a version number to your display:
// Add version number
let infoDictionary = NSBundle.mainBundle();
let version = infoDictionary.objectForInfoDictionaryKey("CFBundleShortVersionString") as! String;
let build = infoDictionary.objectForInfoDictionaryKey("CFBundleVersion") as! String;
self.labelVersion.text = String(format: "v%@.%@", version, build);
Having a version number in the settings can be invaluable when trying to debug customer issues.
Next steps
Add some quality and animations to the counter control
Previous posts in the series
- Plan for DaysLeft App
- Baby Steps using Swift
- Porting UserSettings Code To Swift
- Basic UI in Swift
- Writing a Today Widget in Swift
- Adding a Custom Control
The code for the project is also available on GitHub